- What is Enumerate in Python?
- Python Enumerate Function - Syntax and Parameters
- Primary Functions of Enumerate in Python!
- Different Uses of Enumerate in Python
- Advantages of Using Enumerate Function in Python
- Enumerate in Python - Common Errors and Mistakes
- Useful Tips for Using Enumerate Function in Python
- Conclusion
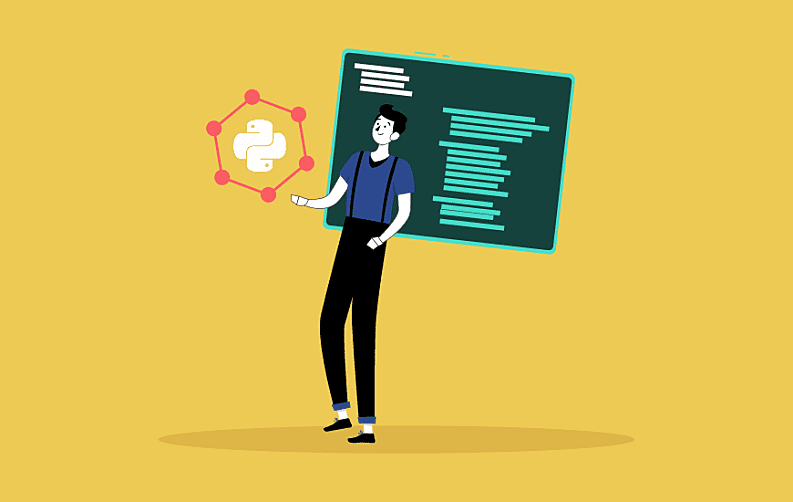
Python is one of the most popular programming languages in the current IT landscape. The primary reason behind its popularity is that it can easily integrate with modern technologies like data science, artificial intelligence, etc. Albeit that, there are multiple functions in Python that deal with multiple use cases. For example, Python for mobile app development, web development, web apps, etc.
One similar built-in function is ‘enumerate’ in Python. In this article, we will explain the different facets of Python enumerate function. Facets such as the syntax, the parameters, uses and the different examples associated with the Python function enumerate. So, let’s learn!
What is Enumerate in Python?
Enumerate in Python is a built-in function. It helps the user iterate over an iterable. For example, list, tuple, or string. This helps in keeping track of both the index (position) and the value of each iterable item:
To better understand, here is a breakdown of what the enumerate function in Python does:
- Takes an Iterable: In this, the user provides a sequence of elements for a list or a string. This is taken as an input to the enumerate function.
- Adds a Counter: Adding a counter helps essentially with the iterator. It adds a counter that starts from a specified value (by default, 0) to each element in the iterable.
- Return Tuples: During each iteration, an enumerate object returns tuples that contain two elements:
- The current index (counter) from the iterable.
- A corresponding value (element) from the iterable.
Python Enumerate Function - Syntax and Parameters
Starting with any function requires the user to understand its syntax and associated parameters. For enumerate, they are:
Syntax:
enumerate(iterable, start=0)
Parameters:
- iterable (required): It is a sequence used by the user to iterate Python enumerate list, tuple, or string.
- start (optional): It is an integer that specifies the starting value of the index counter. However, the default value is set to 0.
Return Value:
Each tuple contains two elements: enumerate returns and enumerate objects. It is used as an iterator that yields tuples for each item in the sequence.
- The first element is the index position of the item.
- The second value is the value of the item.
Example:
fruits = ["apple", "banana", "cherry"]
for index, fruit in enumerate(fruits):
print(f"Index: {index}, Fruit: {fruit}")
This code will output:
Index: 0, Fruit: apple
Index: 1, Fruit: banana
Index: 2, Fruit: cherry
Key Points:
- Enumerate is used when the user wants to process an item that is based on both value and position within a sequence.
- Unpacking returned tuples into separate variables for index and value improves code readability.
- Enumerate can be used to customize the starting index for a counter that uses the ‘start’ parameter.
Primary Functions of Enumerate in Python!
There are several primary functions of enumerate in Python. To throw light on them, they are:
1. Iterate with index and value
fruits = ["apple", "banana", "cherry"]
for index, fruit in enumerate(fruits):
print(f"Index: {index}, Fruit: {fruit}")
In this, the code iterates over the fruit lists and prints the index & fruit for each item. To this, the enumerate function in Python adds a counter to each item available in the list. This index starts from 0. After that, the loop unpacks the tuples that are returned by enumeration in two variables, i.e., index and fruit.
2. Start index at 1
fruits = ["apple", "banana", "cherry"]
for index, fruit in enumerate(fruits, start=1):
print(f"Index (start 1): {index}, Fruit: {fruit}")
This code iterates over the list of ‘fruits’. However, this time the start parameter of the enumerate is set to 1. So, the counter starts at 1 instead of 0.
Index | Fruit |
---|---|
0 | apple |
1 | banana |
2 | Cherry |
Index (start 1) | Fruit |
1 | apple |
2 | banana |
3 | cherry |
3. Enumerate a list of Objects
Let’s start with the object “Cars” below:
Class Cars:
The objective here would be to iterate the object “Cars” and print out the names along with the index. To do that we will use the enumerate in Python function. Have a look at the code below:
Car_Company = [Cars(‘Bentley’), Cars(‘Mercedes’), Cars(‘Audi’)]
For index, Cars in enumerate(Car_Company):
print(index, Cars.Car_Name)
Output:
0 Bentley
1 Mercedes
2 Audi
4. Enumerating the list of Tuples
Now, let’s take the example of a tuple representing a dataset. In this tuple, the first element is a label and the remaining are the data points. The task here is to iterate the list and print out each and every label that corresponds to its data points. To do this, we can use the enumerate function. Let’s see the code first:
Student_Age = [(‘class A age’, 10, 11, 10), (‘class B age’, 12,10,11), (‘class C age’, 10, 10, 11)]
For index, (labe, *Student_Age) in enumerate(Student_Age):
print(index, label, Student_Age)
Output:
0 class A age [10, 11, 10]
1 class B age [12, 10, 11]
2 class C age [10, 10, 11]
5. Enumerating a dictionary
Now, let’s suppose we have a dictionary of words that are mapped from words to their corresponding frequency counts. To iterate the dictionary and print out each and every word along with its frequency count, we can use the enumerate function. Let’s check the code for that:
Word_counts = {‘Bentley’:3, ‘Mercedes’:2, ‘Audi’:1}
For index, (word, count) in enumerate(word_counts.items()):
print(index, word, count)
Output:
0 Bentley 3
1 Mercedes 2
2 Audi 1
Different Uses of Enumerate in Python
There are several use cases associated with the enumerate function. Let’s explore:
1. Using Python enumerate for loops:
It is one of the more common uses of the enumerate function in Python. It helps unpack tuples returned by enumerate in your loop. This helps you easily access both the index and the value of each element simultaneously:
Here's a Python enumerate example for a loop:
fruits = ["apple", "banana", "cherry"]
for index, fruit in enumerate(fruits):
print(f"Index: {index}, Fruit: {fruit}")
This code will print:
Index: 0, Fruit: apple
Index: 1, Fruit: banana
Index: 2, Fruit: cherry
2. Optional starting index:
The enumerate function allows the user to specify an optional starting value for the index. For that, it uses the ‘start’ parameter. For instance, to start the counter at 1:
for index, fruit in enumerate(fruits, start=1):
print(f"Index: {index}, Fruit: {fruit}")
This will print:
Index: 1, Fruit: apple
Index: 2, Fruit: banana
Index: 3, Fruit: cherry
3. map function:
Map function in Python applies a function to each item in an iterable. Here, enumerate can be used to provide both the index and the value to the function used with map:
Here is an example:
numbers = [1, 2, 3, 4]
def square(index, num):
return index, num * num
result = map(square, enumerate(numbers))
print(list(result)) # Output: [(0, 1), (1, 4), (2, 9), (3, 16)]
4. Python String Split():
Python String split() breaks a string into a list based on a separator. For this, enumerate can be used to get the index of each word while splitting.
Example for String split():
sentence = "This is a sample sentence"
for index, word in enumerate(sentence.split()):
print(f"Index: {index}, Word: {word}")
Output:
Index: 0, Word: This
Index: 1, Word: is ... (and so on)
5. Python Multithreading:
While enumerate doesn’t directly impact Python multithreading, it can be used to assign unique identifiers for tasks within threads. Basically, it can be used for tracking and managing these identifiers.
Here’s an example:
import threading
def task(index, data):
# Process data using the index for identification
print(f"Thread {index} processing data: {data}")
data_list = [10, 20, 30]
threads = []
for i, data in enumerate(data_list):
thread = threading.Thread(target=task, args=(i, data))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
6. defaultdict in Python:
defaultdict in Python provides a default value for the missing keys. Enumerate can be used to create a dictionary to know where the index becomes the key and the value can be the item itself.
Here is the example:
from collections import defaultdict
numbers = [1, 2, 3, 2, 1]
# Create a dictionary with count of each number
number_counts = defaultdict(int)
for index, num in enumerate(numbers):
number_counts[num] += 1
print(number_counts) # Output: defaultdict(<class 'int'>, {1: 2, 2: 2, 3: 1})
Advantages of Using Enumerate Function in Python
There are several advantages of using the enumerate function in Python compared to traditional loops. These are:
1. Simplified Index Tracking:
It eliminates the need to manually manage a separate index variable within the loop. The function automatically assigns an index to each item available in your Python enumerate list. This reduces boilerplate code and potential errors that are associated with manipulating the indexes manually.
2. Enhanced Readability:
If you want to unpack the output of enumerate into separate variables for index and value, your code is explicit about the working of each step. In simpler words, any guy could read the code and understand what it is meant to do. This helps in improving code clarity and maintaining yourself and others.
3. Avoiding Off-By-One Errors:
Manually managing the indexes can be error-prone. This is especially true if you are dealing with zero-based indexing in Python. With enumerate, you always have the correct associated index with each item. This reduces the possibility of off-by-one errors that cause unexpected behavior.
4. Memory Efficiency:
Enumerate is a lightweight function. So, the iterator object it creates doesn’t consume extra memory for storing indices. This can be beneficial while working with large datasets.
Enumerate in Python - Common Errors and Mistakes
While implementing the enumerate function in Python, there are some important things to keep in mind that create some common errors and mistakes.
Common errors while using Enumerate in Python:
- While enumerate works with lists, tuples, and strings, it is not ideal for sets if you want to process elements in a specific order.
- The use of non-numeric values or a very large number can lead to unexpected behavior or errors.
- Forgetting to unpack the tuple that is returned by enumerate()
- Using an out-of-range index value.
- Changing the length of the sequence that is being enumerated during the iteration.
- Assuming that the iteration order is consistent for custom classes.
- Not creating a copy before iterating or taking a different approach like list comprehension.
Ways to avoid these errors!
- Make sure to unpack the tuple returned after the enumerate() function has been executed.
- Always use the len() function to figure out the length of the sequence and run out of range.
- Don’t change the length of the sequence during iterations.
- Choose clear variable names like indexes and items that will improve code readability and reduce confusion.
- Explore alternative dictionaries with numerical keys or custom classes that track indices.
Useful Tips for Using Enumerate Function in Python
Before jumping onto a Python enumerate list or using the Python enumerate for loop, it is essential to understand useful tips to use it effectively. So, here they are:
1. Tracking Index and Value:
With enumerate, the user can iterate over a sequence for both the index and value of each item. So, the function is particularly useful in times of performing an operation that depends on both the element and its position.
2. Customize Starting Index
Enumerate optionally can be used to specify a starting index for the counter. So, for instance, if you want the first index to be 1 for human readability then it can start from 1.
3. Unpack for Readability
While using Python enumerate for loop, it is a common practice to unpack returned tuples into separate variables. This is for both the index and the value. It improves code readability by making it clear each variable is represented within the loop body.
4. Iterate over Strings
Enumerate not only works with lists and tuples but also with strings. It allows you to process characters within a string along with their corresponding positions within the string.
5. Avoid Enumerate with Sets
It is not recommended to use enumerate with sets. Sets are usually unordered lists, therefore, the value returned may not be consistent. So, if you need to process elements in a specific order, convert these sets to lists or tuples before using enumerate.
By using these tips, you can easily leverage enumerate to write cleaner, more efficient, and easy-to-understand Python code. This can help deal with sequences and their associated element positions.
Conclusion
A majority of mobile app development companies are using Python simply for the ease of these functions. The enumerate in Python is a useful built-in function that is used for iterating sequences and keeping track of the index. The Python enumerate function can be used in multiple case scenarios that include working with objects, tuples, and dictionaries. There are alternatives, however, it becomes easier to accomplish this task using the function at hand.