- What is an Arrays in Java?
- Types of Array in Java
- How does an Array in Java Program look like?
- Array Declaration in Java
- Arrays in Java - Advantages and Disadvantages
- Significance of Array Data Structure in Java
- What is Datastructure?
- How to Reverse an Array in Java?
- How to Perform Binary Search using Arrays in Java?
- How to Perform Cloning using Java Arrays?
- Wrapping Up!
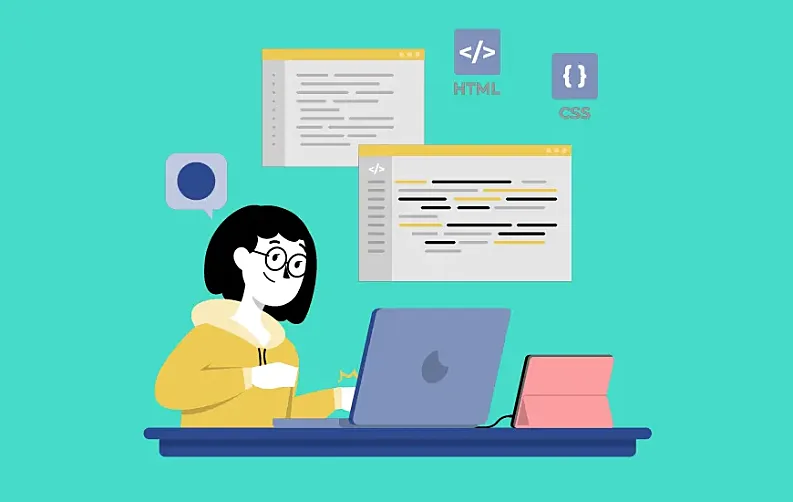
Arrays in Java are amongst the most fundamental concepts that programmers need to learn. It is essential because almost every programmer needs to get a hold of it. It is because arrays have a much wider application than one can anticipate. Therefore, it becomes essential to learn the concept of arrays.
In this article, we have tried to provide a basic understanding to develop your own array program in Java.
What is an Arrays in Java?
To simply define arrays in Java, an array is a single variable declared in Java that holds multiple values.
For instance, a single variable holding a value in Java would look like this:
int num = 23;
However to define an array in Java or declare a new array in Java, it would look something like this:
int num[ ] = {1,2,3,4,5,6};
This is another example for storing words in java programs on strings and arrays:
string Cars[ ]= {Lamborghini, Ferrari, Mercedes, Mustang, Volkswagen};
The idea behind arrays in Java is to create datasets of similar datatype and with similar metadata.
Types of Array in Java
There are several types of arrays in Java that can be used for holding values that are numeral, alphabetical, and decimal in nature. To do that there are multiple datatypes that can be used to initialize the data in a variable.
Note: For ease of understanding and visualizing the array, we’ll use the example X, Y, and Z axis.
The different types of arrays in Java are:
1. One Dimensional Array (1-D)
A one-dimensional array is linear in nature. It means the data stored in a one-dimensional array lies on a single axis. In this case, you can imagine that the data is stored on X-axis.
An example of one-dimensional array would be:
int arr[ ]= {2,4,3,6,5,7,};
2. Two Dimensional Array (2-D)
A 2 dimensional array in Java lies on two axis. For ease of understanding, consider them X and Y. Another way of understanding 2 dimensional arrays in Java is to understand them as data declared in the form of rows and columns.
Here is a two dimensional array in Java with example:
int num [3 ] [ 3]= {{1,2,3},{2,3,4},{3,4,3}};
3. Three Dimensional Array (3-D)
A three dimensional array will be laid on 3 axis i.e. X, Y, and Z. This can be divided into blocks, rows, and columns. A three dimensional if you can imagine looks like a 3D cube.
Example of three dimensional array would be:
int num [ ][ ][ ] = {{{1,2,3}}, {{1,2,3}}, {{2,3,1}},{{1,2,3}}, {{1,2,3}},{{2,3,1}}, {{1,2,3}}, {{1,2,3}}, {{2,3,1}}};
How does an Array in Java Program look like?
Here’s an example of how an array program in Java look like:
The example above prints the integer stored in the array.
Array Declaration in Java
Considering that there are three types of arrays in Java. Below are the ways you can declare an array in Java.
1. One Dimensional Array:
The array declaration in Java for a one dimensional array would look like:
int num[5]={1,2,3,4,5};
A) int:
Here “int” is used for declaring the datatype. For instance, if we use “string” in place of “int” then the data inside the array would be considered as a string. The value of a string is pre-established based on ASCII (American Standard Code for Information Interchange) codes. If two strings are compared then the ASCII value of each string is compared with the other one. It is possible because in the case of a string, the string used as the value has its own index, for example, “words”. The index value of “w” in “words” would be 0 while the value of “s” would be “4”.
Note: The count for the index starts from 0 in every case.
B) num[5]:
“Num” is the name of the variable through which this array would be identified. The number “5” inside the square bracket describes the length of the array. If we are providing the value of the array in the program itself, there is no explicit need for describing the length beforehand. However, if you are taking input from the user, describing the value beforehand would be ideal.
C) {1,2,3,4,5}:
This is the data inside the array that has been input into the array beforehand in the program. In order to take the input from the user, the programmer is required to use the input function along with a loop.
2. Two Dimensional Array:
The declaration of 2 dimensional array in Java would look like this:
Int num[5 ] [5 ]={{1,2,3,4,5},{6,7,8,9,10}}
num[5][5]:
Here the digits “5” and “5” is the length of the rows and column. Visually, the data stored inside the array would look like the table below:
These values are accessed in an array using their index values. For instance, the index value of the data “1” would “0,1”, the index value of the data “5” would be “4,4”, the index value of “8” would be “3,2”
3. Three Dimensional Array:
Below is the example of three dimensional array declaration in Java:
Int num [3 ][ 3][3 ] = {{{1,2,3}}, {{1,2,3}}, {{2,3,1}},{{1,2,3}}, {{1,2,3}},{{2,3,1}}, {{1,2,3}}, {{1,2,3}}, {{2,3,1}}};
num[3][3][3]:
Here the value inside the square brackets “3”,”3”, and “3” describes the block, rows, and columns in the same order as stated here.
Note: The visualization of a 3D array in a 2D space would look similar to a 2 dimensional array in Java. However, it is not possible to showcase how the values are stored in three dimensional array in Java.
Arrays in Java - Advantages and Disadvantages
Just like any attribute or property has any advantages and disadvantages in a programming language. Quite similarly even arrays in java have its own advantages and disadvantages. These are:
Advantages
They can be easily declared and its elements can be accessed via indexes with ease. Memory utilized by an array is contiguous in nature making it easily accessible in nature. It allows the storage of different types of data types. Arrays are very easy to traverse for accessing elements. Size of the array is dynamic making it suitable for changing the size of it at any time.
Disadvantages
Arrays are flexible in size. However, if you allocate a size while declared it can’t be changed later on. An array can only hold data for which it is declared. Arrays require memory allocation during the time of declaration which can not be changed later on. Arrays don’t have any built-in operations such sorting, searching, etc.
Significance of Array Data Structure in Java
To understand the significance of an array and the relation between an array & data structure, it is important to understand what data structure actually means.
What is Datastructure?
A data structure in essence is a format for storing data. The idea behind forming a data structure is to organize, process, store, and retrieve it with ease. Every data structure follows a logic and provides the capability to store and use that data with that logic. One of the most common examples of it if you are aware would be stack and queue.
1 | 3 | 4 | 4 | 7 | 6 | 2 | 8 | 1 | 0 | 9 |
Now, if we have an array then one can understand that it is in itself a linear data structure. An array in essence is nothing but a way to store similar data using a single variable used for its identification. Another interesting aspect is that the datatype remains the same for each array and the variable using which it is initialized. For instance, int stands for integer, string is used for both alphabets and digits, boolean is for binary, etc.
How to Reverse an Array in Java?
There are several methods to reverse an array in Java. It is important because many times, it is required to access the data from its last index. When these concepts are applied on an advanced level, use cases such as this help in sorting, searching, and finding the data with ease.
1st Method: Printing in the Reverse Order
In this method to reverse an array in Java, we use the length property to access all the indexes in the array to access all the data stored in it. After that, we run a reverse loop from the last index and print the result out.
2nd Method: Reversing an array and ArrayList in Java
In this, we are creating a reverse function explicitly. The logic in this function is created such that once an array is passed on to it, it reverses the values of the array and returns the value to the code when it was called.
3rd Method: Utilizing the loop for reversing the array
In this method similar to the previous one, we create a function name “reversed”. In the main method, we declare an array with the value. After that, we calculate the length by using the length attribute and then call the function.
In this function, we declare a new array and run a loop in reverse to store the values in the original array in reverse order. Once that is laid out, we print the values using another “for” loop.
How to Perform Binary Search using Arrays in Java?
Binary search is an algorithm that is used for searching an element in an array. Binary search is performed by sorting the elements in ascending order and then dividing the array in two parts. Once the array is divided, it starts searching for required elements from the middle. This thereby helps in reducing the time of search.
Below, we have provided the code for binary search in java:
class BinarySearch{
public static void binarySearch(int array[], int first, int last, int key){
int middle = (first + last)/2;
while( first <= last ){
if ( array[middle] < key ){
first = middle + 1;
}else if ( arr[middle] == key ){
System.out.println("Element is found at index: " + middle);
break;
}else{
last = middle - 1;
}
middle = (first + last)/2;
}
if ( first > last ){
System.out.println("Element is not found!");
}
}
public static void main(String args[]){
int arr[] = {10,20,30,40,50};
int key = 30;
int last=array.length-1;
binarySearch(array,0,last,key);
}
}
How to Perform Cloning using Java Arrays?
Cloning in an array is performed for cloning a source array to a destination array of the same size. This is done using the clone() method. When the clone method is invoked, it references a new array which contains the elements of the source array.
Below is the code of cloning arrays in java:
class Student implements Cloning
{
int rollnumber;
String name;
Student(int rollnumber, String name)
{
this.rollnumber = rollnumber;
this.name = name;
}
@Override public Object cloning()
throws CloneNotSupportedException {
return super.cloning();
}
}
public class CloningDemo
{
public static void main(String[] args)
throws CloneNotSupportedException
{
Student student1 = new Student(101, "John Mayor");
Student student2 = (Student) student1.cloning(); System.out.println("student1: " + student1);
System.out.println("student2: " + student2);
assert student1.rollnumber == student2.rollnumber;
assert student1.name == student2.name;
}
}
Wrapping Up!
Java is one of the most popular and widely used languages in the world. In fact, even the top software development companies in USA use it for a lot of their projects. Learning arrays in Java is almost fundamental to utilizing the programming language to its full potential. It is important to understand because, during advanced applications, enormous streams of data are stored inside it. As a programming student, it is essential to understand the applications of arrays and how they can be utilized well. With this article, our intention was to provide you with a basic understanding to learn arrays in Java. We hope this article may have been of some help to you. Also thank you for reading it until the end.
Frequently Asked Questions
-
What is an array in Java?
An array is a collection of data with a similar data type. It is a form of linear data structure that is used in Java.
-
Who would win between Kotlin vs Java?
-
How to learn array in Java?
-
What are some resources for learning Java array tutorial with examples?
-
What is a multidimensional array in Java?
-
How do I declare and initialize an array in java
-
Can I change the size of an array once it is declared in Java?